보통엔티티에는 해당 데이터의 생성 / 수정시간을 포함하고 있음
-> 차후 유지보수에 있어 굉장히 중요한 정보이기 때문에 있어야함
그러나, 매번 DB에 변화가 있을 때마다 데이터를 생성 / 수정하는 코드를 작성하게 되면 코드가 굉장히 지저분해질 수 있음
(물론 귀찮은것도 크지만 ㅋㅋㅋㅋ)
그래서 이번에는 이것들을 자동으로 해줄 수 있는 기능을 사용해서 코드를 작성해보려함
03_5 JPA Auditing으로 생성 / 수정시간 자동화하기
domain 패키지에 클래스 생성
*BaseTimeEntity
import lombok.Getter;
import org.springframework.data.annotation.CreatedDate;
import org.springframework.data.annotation.LastModifiedDate;
import org.springframework.data.jpa.domain.support.AuditingEntityListener;
import jakarta.persistence.EntityListeners;
import jakarta.persistence.MappedSuperclass;
import java.time.LocalDate;
import java.time.LocalDateTime;
@Getter
@MappedSuperclass //1
@EntityListeners(AuditingEntityListener.class) //2
public class BaseTimeEntity {
@CreatedDate //3
private LocalDateTime createdDate;
@LastModifiedDate //4
private LocalDateTime modifiedDate;
}
이 BaseTimeEntity는 모든 개체(entity)의 상위 클래스가 되어 개체들의 생성 / 수정 시간을 자동으로 관리하는 역할을 함
+
전에 작성했던 Posts 클래스가이 BaseTimeEntity를 상속받도록 변경
*Posts
public class Posts extends BaseTimeEntity {
+
JPA Audting 어노테이션들을 활성화 할 수 있게, application 클래스에 활성화 어노테이션을 추가
*Application
@EnableJpaAuditing // JPA Auditing 활성화
@SpringBootApplication
public class Application {
이제 테스트 코드를 작성!
*PostsRepositoryTest
@Test
public void BaseTimeEntity_register() {
//given
LocalDateTime now = LocalDateTime.of(2023, 4, 17, 0, 0, 0);
postsRepository.save(Posts.builder()
.title("title")
.content("content")
.author("author")
.build());
//when
List<Posts> postsList = postsRepository.findAll();
//then
Posts posts = postsList.get(0);
System.out.println(">>>>>>>>>>>> createDate=" + posts.getCreatedDate()
+ ", modifyDate=" + posts.getModifiedDate());
assertThat(posts.getCreatedDate()).isAfter(now);
assertThat(posts.getModifiedDate()).isAfter(now);
}
}
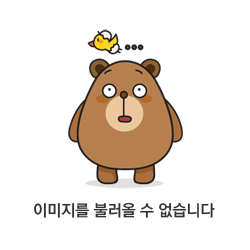
==> Test를 돌려보니, 현재 시간이 잘 나온 것을 볼 수 있었음
==> 이제 앞으로 추가될 개체(entity)들은 BaseTimeEntity만 상속 받으면 자동으로 등록 / 수정일이 관리가 됨
'프로젝트 > 스프링 부트와 AWS로 혼자 구현하는 웹 서비스_실습' 카테고리의 다른 글
스프링부트와 AWS로 혼자 구현하는 웹 서비스 12_AWS (0) | 2023.08.22 |
---|---|
스프링부트와 AWS로 혼자 구현하는 웹 서비스 11 (0) | 2023.08.19 |
스프링 부트와 AWS로 혼자 구현하는 웹 서비스 09 (0) | 2023.08.16 |
스프링 부트와 AWS로 혼자 구현하는 웹 서비스 08 (0) | 2023.08.15 |
스프링 부트와 AWS로 혼자 구현하는 웹 서비스 07 (0) | 2023.08.14 |